This going to be just a summary of what steps is needed to do to implement Facebook login to Flutter for Android, I’ll post my code I use as well so you can use that to login too. The official code I found on Firebase was even little bit outdated so it could come handy.
The steps to get things working from scratch:
- Create a new Flutter project, you can use this command (use your own domain & name): flutter create –org com.yourdomain appname
- Have an Android emulator ready for Flutter through Visual Code or Android Studio
- Create a new Firebase project at https://console.firebase.google.com/
- Create a new Android app in Firebase
- When adding a new Android app, following the steps do add configurations to the C:\…\myapp\android\app\build.gradle and C:\…\myapp\android\build.gradle with the apply plugin, classpath to depenceices and so forth.
- Also don’t forget adding the your json file containing your Firebase details which you can download from Firebase under your app to your C:\…\myapp\android\app\google-services.json
- Create a new app at https://developers.facebook.com/
- Create a new file called strings.xml and add it to your values folder myapp\android\app\src\main\res\values\
You want your file to look something like this:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">com.myappname</string>
<!-- Replace "000000000000" with your Facebook App ID here. -->
<string name="facebook_app_id">000000000000</string>
<!--
Replace "000000000000" with your Facebook App ID here.
**NOTE**: The scheme needs to start with `fb` and then your ID.
-->
<string name="fb_login_protocol_scheme">000000000000</string>
</resources>
You should see your Facebook App ID in the top of your dashboard when created your app like this
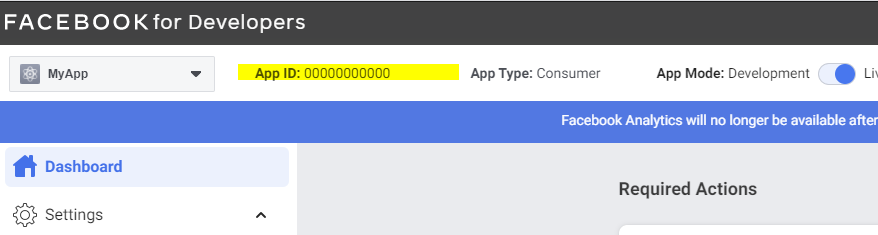
Then you want the fb_login_protocol_scheme which is the exact same thing but with the characters fb before your App ID.
- (following these steps from facebook.meedu.app) Go to your AndroidManifest file at C:\…\myapp\android\app\src\main\AndroidManifest.xml and make sure you have <uses-permission android:name=”android.permission.INTERNET”/>
- Add this metadata inside the <application> element:
<meta-data android:name="com.facebook.sdk.ApplicationId" android:value="@string/facebook_app_id"/>
<activity android:name="com.facebook.FacebookActivity"
android:configChanges="keyboard|keyboardHidden|screenLayout|screenSize|orientation"
android:label="@string/app_name" />
<activity
android:name="com.facebook.CustomTabActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="@string/fb_login_protocol_scheme" />
</intent-filter>
</activity>
- Enable Facebook authentication in Firebase under Authentication -> Sign In methods, and when enabling, enter the App ID and the Secret (the secret is found in your facebook developers app page).
Now we are going to use the flutter_facebook_auth Flutter package, I am using version ^3.5.0.
Add dependencies to your pubspec.yaml file under dependencies:
firebase_core: ^1.3.0
firebase_analytics: ^8.1.2
firebase_auth: ^1.4.1
flutter_facebook_auth: ^3.5.0
You might skip adding analytics if it is not used.
Now you can try it out! Feel free to copy my code:
import 'package:firebase_auth/firebase_auth.dart';
import 'package:firebase_core/firebase_core.dart';
import 'package:flutter/material.dart';
import 'package:flutter_facebook_auth/flutter_facebook_auth.dart';
void main() {
WidgetsFlutterBinding.ensureInitialized();
runApp(App());
}
class App extends StatefulWidget {
// Create the initialization Future outside of `build`:
@override
_AppState createState() => _AppState();
}
class _AppState extends State<App> {
/// The future is part of the state of our widget. We should not call `initializeApp`
/// directly inside [build].
final Future<FirebaseApp> _initialization = Firebase.initializeApp();
@override
Widget build(BuildContext context) {
return FutureBuilder(
// Initialize FlutterFire:
future: _initialization,
builder: (context, snapshot) {
// Check for errors
if (snapshot.hasError) {
print("err!");
return Container();
}
// Once complete, show your application
if (snapshot.connectionState == ConnectionState.done) {
return MyApp();
}
// Otherwise, show something whilst waiting for initialization to complete
return Container();
},
);
}
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'FileIdea Facebook Login',
theme: ThemeData(
// This is the theme of your application.
//
// Try running your application with "flutter run". You'll see the
// application has a blue toolbar. Then, without quitting the app, try
// changing the primarySwatch below to Colors.green and then invoke
// "hot reload" (press "r" in the console where you ran "flutter run",
// or simply save your changes to "hot reload" in a Flutter IDE).
// Notice that the counter didn't reset back to zero; the application
// is not restarted.
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'FileIdea Facebook Login'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key? key, required this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
final FirebaseAuth _auth = FirebaseAuth.instance;
bool isloggedin = false;
@override
void initState() {
super.initState();
_auth.userChanges().listen((event) {
print(event);
updateStatus(event);
});
}
updateStatus(event) {
setState(() {
isloggedin = event != null;
});
}
Future<UserCredential> signInWithFacebook() async {
// Trigger the sign-in flow
final res = await FacebookAuth.instance.login();
// Create a credential from the access token
final facebookAuthCredential =
FacebookAuthProvider.credential(res.accessToken!.token);
// Once signed in, return the UserCredential
return await FirebaseAuth.instance
.signInWithCredential(facebookAuthCredential);
}
@override
Widget build(BuildContext context) {
if (!isloggedin) {
return Scaffold(
appBar: AppBar(
// Here we take the value from the MyHomePage object that was created by
// the App.build method, and use it to set our appbar title.
title: Text(widget.title),
),
body: Center(
// Center is a layout widget. It takes a single child and positions it
// in the middle of the parent.
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Material(
elevation: 5.0,
borderRadius: BorderRadius.circular(30.0),
color: Colors.blue,
child: MaterialButton(
minWidth: MediaQuery.of(context).size.width,
padding: EdgeInsets.fromLTRB(20.0, 15.0, 20.0, 15.0),
onPressed: () {
signInWithFacebook();
},
child: Text("Login with Facebook",
textAlign: TextAlign.center,
style: TextStyle(
color: Colors.white, fontWeight: FontWeight.bold)),
),
),
],
),
), // This trailing comma makes auto-formatting nicer for build methods.
);
} else {
return Scaffold(
appBar: AppBar(
// Here we take the value from the MyHomePage object that was created by
// the App.build method, and use it to set our appbar title.
title: Text(widget.title),
),
body: Center(
// Center is a layout widget. It takes a single child and positions it
// in the middle of the parent.
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Container(
child: Text("Home"),
)
],
),
),
);
}
}
}
I’ve highlighted the code I want to show to you that have importance to the login functionality. We store an boolean variable to keep track if the user is logged in or not which we later on use to display either the login button or the “Home” text. To set this variable, we are are listening to _auth.userChanges(), so whenever the state of the user changes, we run our update function to set the isloggedin to true or false if logged out.
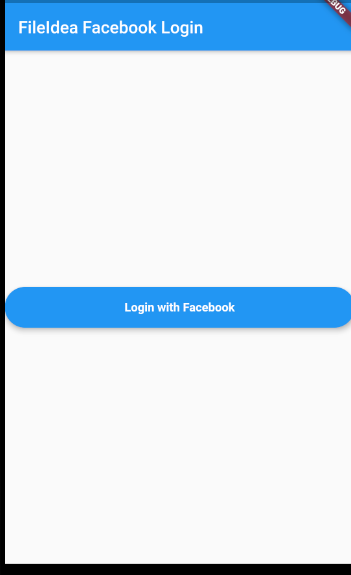
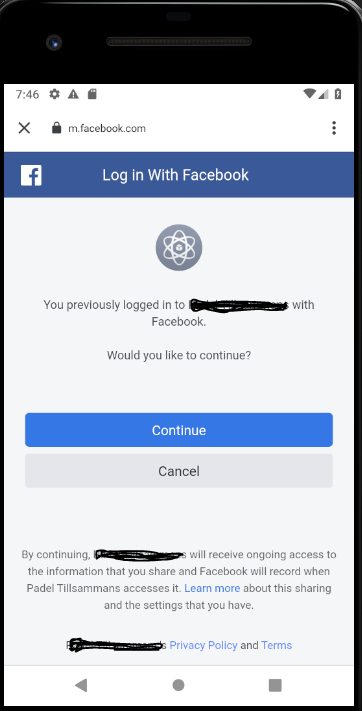
And now Home shows since we are logged in
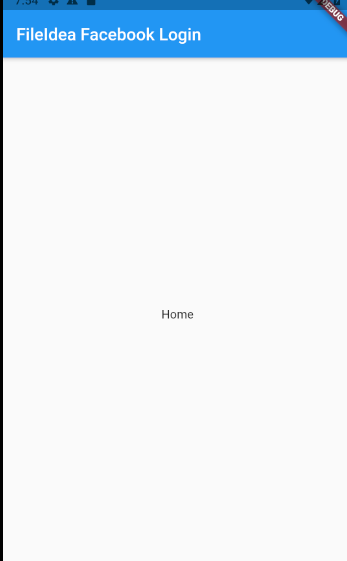
Note that you might need to change minSdkVersion to 25 and add multidex if you have issues building.